Node .js web module
This section describes the .js Web module, and first, you should understand what a Web server is.
What is a Web server?
A Web server generally refers to a Web site server, a program that resides on a certain type of computer on the Internet.
The basic function of a Web server is to provide Web information browsing services. It only needs to support http protocols, HTML document formats, and URLs to work with the client's web browser.
Most web servers support the scripting language (php, python, ruby) on the service side and so on, and get data from the database through the scripting language to return the results to the client browser.
The three most mainstream Web servers are Apache, Nginx, and IIS.
The web app schema
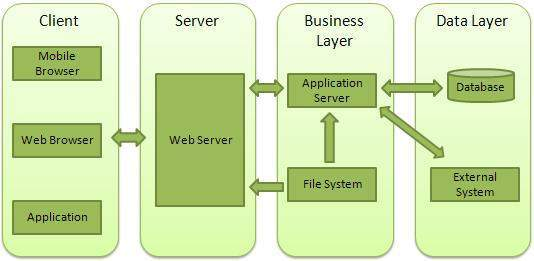
-
Client - Client, generally referred to as a browser, which can request data from the server through the HTTP protocol.
-
Server - The server side, generally referred to as a Web server, can receive client requests and send response data to clients.
-
Business - Business layer, processing applications through a Web server, such as interacting with databases, logic operations, calling external programs, and so on.
-
Data - A data layer that typically consists of databases.
Use Node to create a web server
Node .js provides http modules, http modules are mainly used to build HTTP servers and clients, if you want to use HTTP server or client functions, you must call http modules, the code is as follows:
var http = require('http');
Here's a demonstration of a basic HTTP server architecture (using port 8081) to create .js server file, as follows:
var http = require('http'); var fs = require('fs'); var url = require('url'); // 创建服务器 http.createServer( function (request, response) { // 解析请求,包括文件名 var pathname = url.parse(request.url).pathname; // 输出请求的文件名 console.log("Request for " + pathname + " received."); // 从文件系统中读取请求的文件内容 fs.readFile(pathname.substr(1), function (err, data) { if (err) { console.log(err); // HTTP 状态码: 404 : NOT FOUND // Content Type: text/plain response.writeHead(404, {'Content-Type': 'text/html'}); }else{ // HTTP 状态码: 200 : OK // Content Type: text/plain response.writeHead(200, {'Content-Type': 'text/html'}); // 响应文件内容 response.write(data.toString()); } // 发送响应数据 response.end(); }); }).listen(8081); // 控制台会输出以下信息 console.log('Server running at http://127.0.0.1:8081/');
Next we create an index file in this directory.htm code is as follows:
<html> <head> <title>Sample Page</title> </head> <body> Hello World! </body> </html>
To execute a .js file:
$ node server.js Server running at http://127.0.0.1:8081/
Then we enter and open the address in the browser: http://127.0.0.1:8081/index.htm, as shown in the following image:
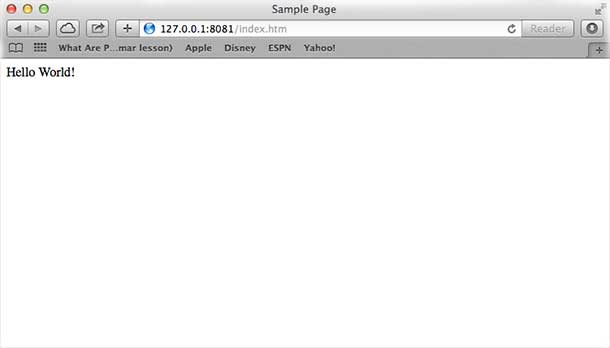
The console output .js the server is as follows:
Server running at http://127.0.0.1:8081/ Request for /index.htm received. # 客户端请求信息
Gif instance demo
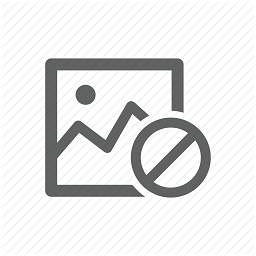
Create a web client with Node
Creating a Web client with Node requires the introduction of the http module to create .js file, as follows:
var http = require('http'); // 用于请求的选项 var options = { host: 'localhost', port: '8081', path: '/index.htm' }; // 处理响应的回调函数 var callback = function(response){ // 不断更新数据 var body = ''; response.on('data', function(data) { body += data; }); response.on('end', function() { // 数据接收完成 console.log(body); }); } // 向服务端发送请求 var req = http.request(options, callback); req.end();
Open a new terminal, perform the .js, the output is as follows:
$ node client.js <html> <head> <title>Sample Page</title> </head> <body> Hello World! </body> </html>
The console output .js the server is as follows:
Server running at http://127.0.0.1:8081/ Request for /index.htm received. # 客户端请求信息
Gif instance demo
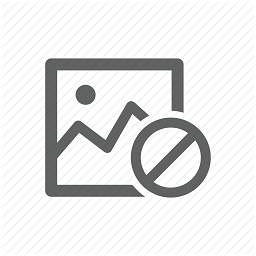