Node .js REPL (Interactive Interpreter)
Node.js REPL (Read Eval Print Loop: Interactive Interpreter) represents a computer's environment, similar to a Window system terminal or Unix/Linux shell, where we can enter commands and receive a response from the system.
REPL's interactive programming environment validates the code you write in real time, making it ideal for validating .js and JavaScript-related APIs.
Node brings its own interactive interpreter to perform the following tasks:
-
Read - Read user input, resolve input Javascript data structure and store it in memory.
-
Execute - Performs the input data structure
-
Print - Output results
-
Loop - Cycle through the above steps until the user presses the ctrl-c button twice to exit.
Node's interactive interpreter is a good way to debug Javascript code.
Start learning REPL
We can start Node's terminal by entering the following command:
$ node >
At this point, we can enter a simple expression and press the enter key to calculate the result.
Simple expression operations
Let's do .js simple math in the command-line window of node and REPL:
$ node > 1 +4 5 > 5 / 2 2.5 > 3 * 6 18 > 4 - 1 3 > 1 + ( 2 * 3 ) - 4 3 >
Use variables
You can store the data in variables and use it when you need it.
Variable declarations need to use the var keyword, which is printed directly if you do not use the var keyword variable.
Variables that use the var keyword can use console .log () to output variables.
$ node > x = 10 10 > var y = 10 undefined > x + y 20 > console.log("Hello World") Hello World undefined > console.log("www.w3cschool.cn") www.w3cschool.cn undefined
A multi-line expression
Node REPL supports entering multi-line expressions, which is a bit like JavaScript. Let's perform a do-while loop:
$ node > var x = 0 undefined > do { ... x++; ... console.log("x: " + x); ... } while ( x < 5 ); x: 1 x: 2 x: 3 x: 4 x: 5 undefined >
... The three-point symbol is automatically generated by the system, and you can return to the line. Node automatically detects whether it is a continuous expression.
Underscore the variable
You can get the results of an expression using underscores:
$ node > var x = 10 undefined > var y = 20 undefined > x + y 30 > var sum = _ undefined > console.log(sum) 30 undefined >
REPL command
-
ctrl s c - exit the current terminal.
-
Ctrl sc pressed twice - exit Node REPL.
-
ctrl s d - Exit Node REPL.
-
Up/Down key - View the history command you entered
-
tab key - lists the current command
-
.help - Lists the usage commands
-
.break - Exit the multi-line expression
-
.clear - Exit a multi-line expression
-
.savename - Save the current Node REPL session to the specified file
-
.loadname - Loads the file contents of the current Node REPL session.
Stop REPL
As mentioned earlier, you can exit rePL by pressing ctrl plus c twice:
$ node > (^C again to quit) >
Gif instance demo
Next, let's demonstrate the instance operation with the Gif diagram:
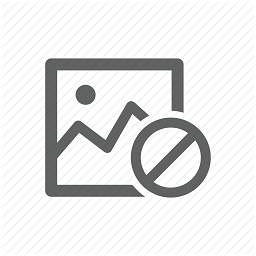